2. VCLとFMXで使う
フォームをまず作ります。
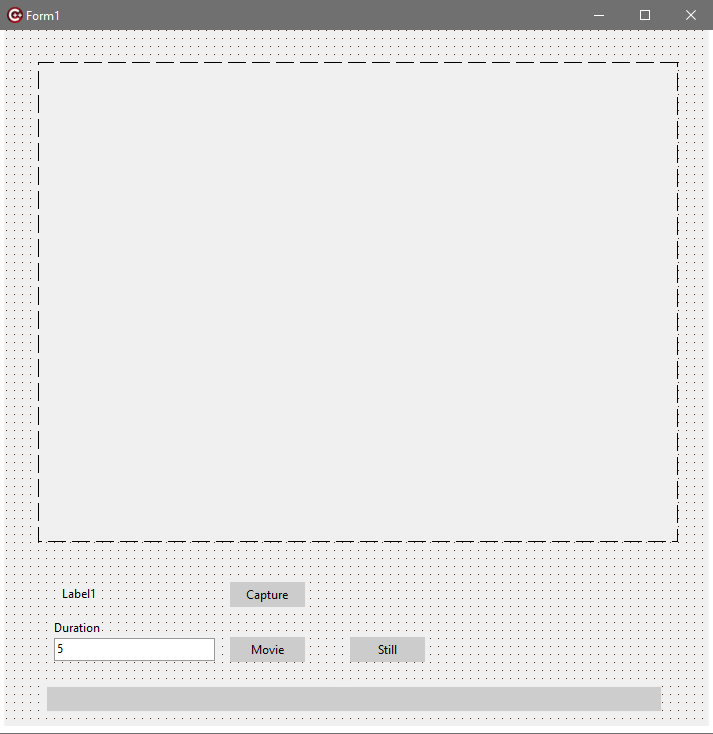
こんな感じで、TImageとTLabelを各1個、TButtonを3個、TLabeledEditを一つ、TProgressbarを一つ配置します。3個のボタンは、それぞれ静止画を画面に表示させる“Capture”、動画を撮影するための“Movie”、静止画をファイルとして記録するための“Still”としています。LabeledEdit内の数字は秒単位の動画記録時間です。ProgressBarは動画や静止画をPC側にダウンロードする際にその経過を示すのに使います。Unit1.cppは、
#include <vcl.h>
#pragma hdrstop
#include "cameralib.h"
#include <jpeg.hpp>
#include "Unit1.h"
//---------------------------------------------------------------------------
#pragma package(smart_init)
#pragma resource "*.dfm"
TForm1 *Form1;
Camera* camera;
extern int filecount;
int Live=0;
EdsError EDSCALLBACK ProgressFunc(EdsUInt32 inPercent, EdsVoid* inContext, EdsBool* outCancel)
{
Form1->TransferProgress->Position = inPercent;
return EDS_ERR_OK;
}
void UpdateProgressBar(unsigned long value)
{
Form1->TransferProgress->Position = value;
}
//---------------------------------------------------------------------------
__fastcall TForm1::TForm1(TComponent* Owner)
: TForm(Owner)
{
camera = new Camera();
if( camera->TotalCameraCount > 0 ){
Label1->Caption = camera->GetName();
Movie->Enabled = true;
Still->Enabled = true;
}
else
Label1->Caption = "no cameras found";
}
//---------------------------------------------------------------------------
void __fastcall TForm1::MovieClick(TObject *Sender)
{
camera->RecordMovie();
Sleep(1000*(StrToInt(Duration->Text)+1));
camera->StopMovie();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::StillClick(TObject *Sender)
{
camera->TakePictureToFile();
}
//---------------------------------------------------------------------------
void __fastcall TForm1::CaptureClick(TObject *Sender)
{
void* data;
long size;
filecount = 1;
TMemoryStream* ImageStream = new TMemoryStream();
camera->TakePictureToStream();
data = camera->getbuffer();
size = camera->getsize();
ImageStream->WriteBuffer(data,size);
ImageStream->Position = 0;
Image1->Picture->LoadFromStream(ImageStream);
delete ImageStream;
}
//-------------------------------------------------------------------------
です。ProgressBarの更新のためにCallBack関数を定義しています。またダウンロードするファイル数を指示するために、int filecountを使います。これらの関数や変数はCameralibの外部で用意する必要があります。そのため若干ごちゃしてますが、やっていることの割には極めて簡潔なコードとなっています。これで動きます。プロジェクトの構成は、
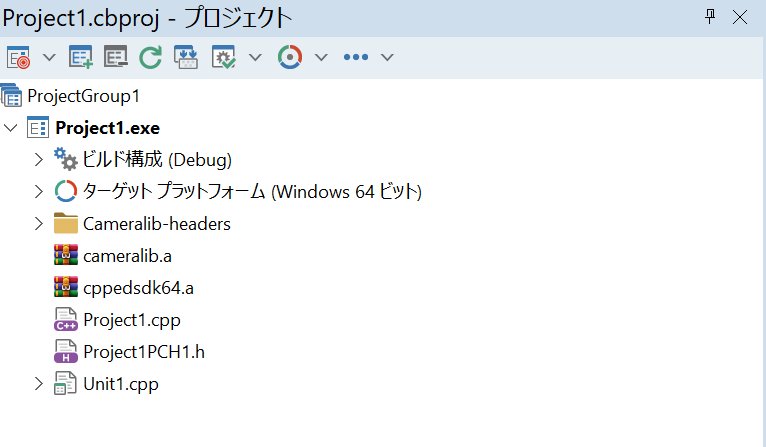
となっていますが、cameralib.a,cppedsdk64.aさらにはCameralib-headersについては、続編で作ります。Cameralib-headersのファイル構成は、
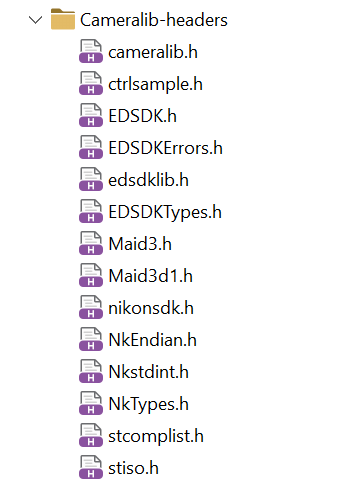
で、こちらから供給するヘッダー以外のファイルはCanonやNikonのサイトから読者にダウンロードしていただくことになります。こちらから供給するのはcameralib.h,edsdklib.h,stcomplist.h,stiso.hのみです。ダウンロードの具体的な方法については、続編で述べます。実行している様子は、
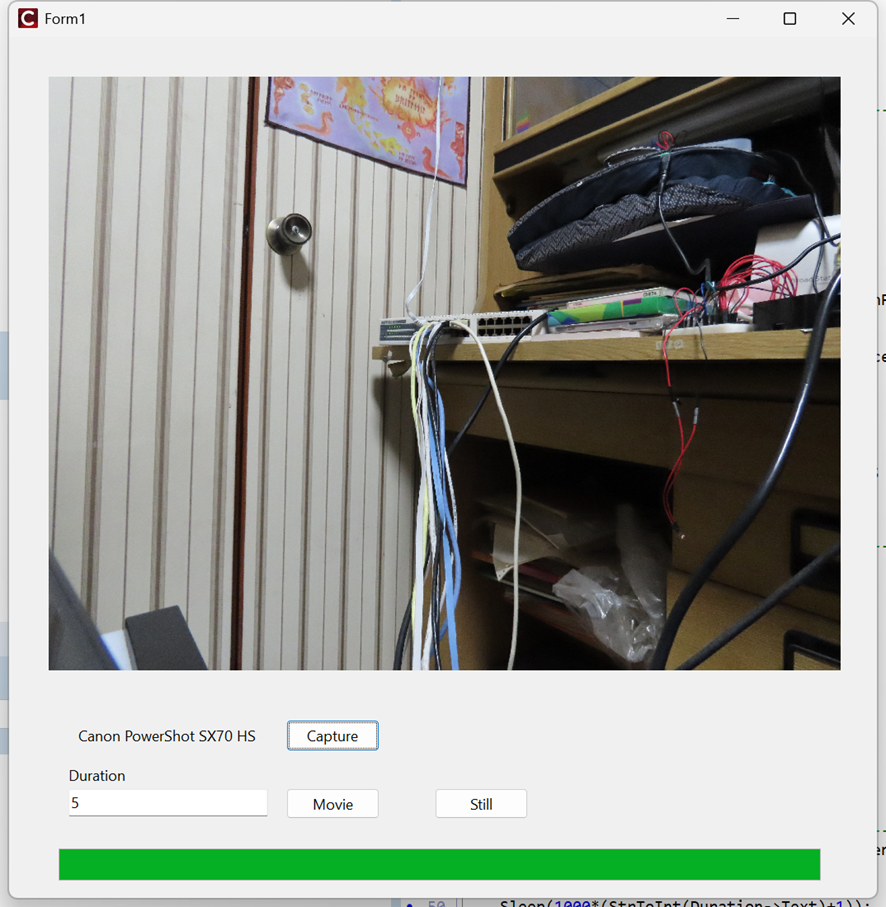
こんな感じです。VectorにアップしているCamera Remote Controlのパネルは、
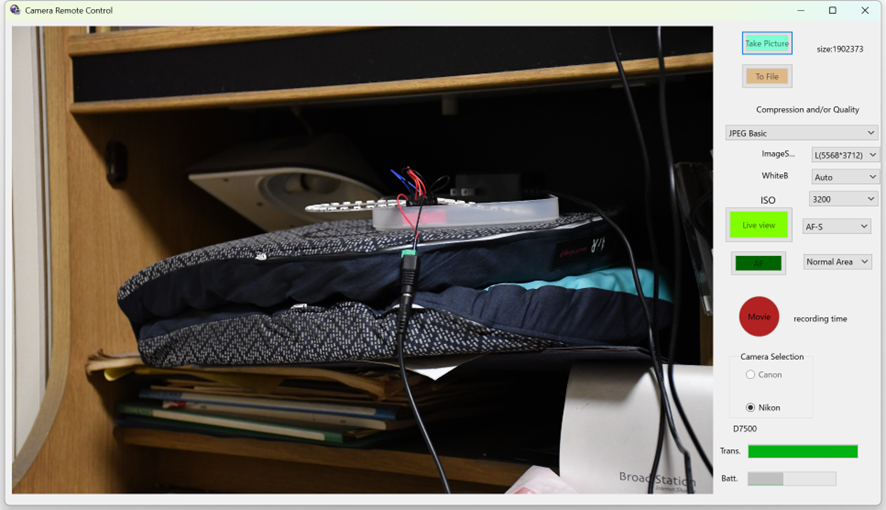
こうなのでかなり簡素ですね。FMX版の場合は、同様なパネルでいいのですが、コードの方では、TImageへの描画が少しだけ違います。
Image1->Bitmap->LoadFromStream(ImageStream);
になります。他には、
EdsError EDSCALLBACK ProgressFunc(EdsUInt32 inPercent, EdsVoid* inContext, EdsBool* outCancel)
{
Form1->TransferProgress->Value = inPercent;
return EDS_ERR_OK;
}
void UpdateProgressBar(unsigned long value)
{
Form1->TransferProgress->Value = value;
}
となります。
コメント