画像ファイルのeximデータの”撮影日時”を取り出すプログラムを書いたよ
VCLやらFMXでエクスプローラーからフォームへのファイルのドラグアンドドロップを実現するためのやり方を3通り示しましたが、2番目の方法でjpegファイルをドラグアンドドロップすると撮影日時を表示するものを作りました。元のプログラムは、これC++ Builder CE tipsです。
車輪の再発明をするのも無駄なので、一通りググりましたが、特に高速に処理する観点で引っかかるものが無かったので、自作しました。ただしeximデータ自体については、元祖の定義書あるいはこれhttp://dsas.blog.klab.org/archives/52123322.htmlが参考になりました。後者のデータ構造を参考にしました。(★が重要でした。)ストレージ内の全てのjpegファイルをスキャンして撮影日時を拾い出してDBを作るという野望がありますから、高速処理が必要であり。画像ファイル全部を一旦読み込む等というのはもってのほかです。
さて、コードを示しますかね。まずUnit1.hは、
#ifndef Unit1H
#define Unit1H
//---------------------------------------------------------------------------
#include <System.Classes.hpp>
#include <FMX.Controls.hpp>
#include <FMX.Forms.hpp>
#include <FMX.Controls.Presentation.hpp>
#include <FMX.Memo.hpp>
#include <FMX.Memo.Types.hpp>
#include <FMX.ScrollBox.hpp>
#include <FMX.StdCtrls.hpp>
#include <FMX.Types.hpp>
//---------------------------------------------------------------------------
class TForm1 : public TForm
{
__published: // IDE で管理されるコンポーネント
TMemo *Memo1;
TLabel *Label1;
TLabel *Label2;
private: // ユーザー宣言
public: // ユーザー宣言
__fastcall TForm1(TComponent* Owner);
virtual void __fastcall DragOver(const Fmx::Types::TDragObject &Data, const System::Types::TPointF &Point, Fmx::Types::TDragOperation &Operation);
virtual void __fastcall DragDrop(const Fmx::Types::TDragObject &Data, const System::Types::TPointF &Point);
};
//---------------------------------------------------------------------------
extern PACKAGE TForm1 *Form1;
//---------------------------------------------------------------------------
#endif
フォームにTMemo,TLabelx2を貼り付けてください。次にUnit1.cppは
#include <fmx.h>
#pragma hdrstop
#include "Unit1.h"
//---------------------------------------------------------------------------
#pragma package(smart_init)
#pragma resource "*.fmx"
TForm1 *Form1;
#define SOI 0xFFD8
#define EOI 0xFFD9
#define APP1 0xFFE1
TFileStream* fp;
bool BigEndian;
//---------------------------------------------------------------------------
void Read16(TFileStream* p,unsigned short* k)
{
unsigned char u,l;
p->Read(&u,1);
p->Read(&l,1);
*k = u*0x100 + l;
}
void BinDump_Buffer(Byte* start, unsigned length)
{
Byte it;
Byte* p;
char obuf[100];
int i;
UnicodeString str;
UnicodeString sub;
p = start;
for( i = 0 ; i < length ; i++ ){
sub.sprintf(L"%02x ",*p++);
str += sub;
}
}
void Dump_Buffer(Byte* start, unsigned length)
{
Byte it;
Byte* p;
char obuf[100];
int i;
p = start;
for( i = 0 ; i < length ; i++ ){
it = *p++;
if( it == 0x0 )
obuf[i] = '0';
else
obuf[i] = it;
}
obuf[i] = 0x0;
}
void Detect_Endian(Byte* start, unsigned length)
{
unsigned char *p = start;
BigEndian = false;
if( *p == 'M' && *(p+1) == 'M' )
BigEndian = true;
else if( *p == 'I' && *(p+1) == 'I' )
BigEndian = false;
else
ShowMessage("Endian error");
if( !BigEndian )
Form1->Label2->Text = "Little_Endian";
else
Form1->Label2->Text = "Big_Endian";
return;
}
unsigned conv(Byte* p)
{
unsigned retval = 0;
if( BigEndian )
retval = *p*0x100000 + *(p+1)*0x10000 + *(p+2)*0x100 + *(p+3);
else
retval = *(p+3)*0x100000 + *(p+2)*0x10000 + *(p+1)*0x100 + *p;
return retval;
}
void IFD_Dump(Byte* address)
{
Byte* start;
char *p;
struct a_tag {
unsigned short tag;
unsigned short tag_type;
unsigned count;
unsigned val2;
} tags[100];
unsigned short ntag;
UnicodeString o;
UnicodeString o2;
start = address;
address += 8;
if(BigEndian ){
ntag = 0x100*(*address);
ntag += *++address;
}
else {
ntag = *address++;
ntag += 0x100*(*address);
}
address++;
o.sprintf(L"%u",ntag);
for( int i= 0; i < ntag ; i++ ){
if( BigEndian ){
tags[i].tag = 0x100*(*address);
tags[i].tag += *++address;
}
else{
tags[i].tag = *address++;
tags[i].tag += 0x100*(*address);
}
address++;
if( BigEndian ){
tags[i].tag_type = 0x100*(*address);
tags[i].tag_type += *++address;
}
else {
tags[i].tag_type = *address++;
tags[i].tag_type += 0x100*(*address);
}
address++;
tags[i].count = conv(address);
address += 4;
tags[i].val2 = conv(address);
if( tags[i].tag == 306 && tags[i].tag_type == 2 ){ // should get null terminated string
p= (char*)(start + tags[i].val2);
o2 = UnicodeString(p);
Form1->Memo1->Lines->Add(o2);
}
address += 4;
}
}
void Parse_Exim(Byte* buffer)
{
Byte* ptr;
Dump_Buffer(buffer,6); // "Exif"\0\0
Detect_Endian(buffer+6,2); //TIFF header "MM" or "II"
BinDump_Buffer(buffer+8,2); // TIFF code 002a
BinDump_Buffer(buffer+10,4); // pointer to oth IFD
IFD_Dump(buffer+6);
}
void Parse_File(UnicodeString filename)
{
Form1->Memo1->Lines->Add(filename);
Form1->Label1->Text = filename;
unsigned short sig;
UnicodeString out,out2;
Byte* buffer;
fp = new TFileStream(filename, fmOpenRead);
if( !fp ){
ShowMessage("file not found");
Application->Terminate();
}
Read16(fp,&sig);
out.sprintf(L"%04X",sig);
if( sig != SOI ){
ShowMessage("sig error ");
return;
}
//unsigned short sig;
unsigned short length;
UnicodeString output;
int i = 0;
do {
Read16(fp,&sig);
output.sprintf(L"%d %04X",i++,sig);
Read16(fp,&length);
output.sprintf(L"length %04X",length);
if( sig == APP1 ){
buffer = new Byte[length];
if( !buffer ){
ShowMessage("can't allocate memory");
Application->Terminate();
}
fp->Read(buffer,length);
Parse_Exim(buffer);
break; // exim block found
}
fp->Seek(length-2,soFromCurrent);
}while( sig != EOI && i < 10 );
delete buffer;
delete fp;
}
//---------------------------------------------------------------------------
void __fastcall TForm1::DragOver(const Fmx::Types::TDragObject &Data, const System::Types::TPointF &Point, Fmx::Types::TDragOperation &Operation)
{
TForm::DragOver(Data,Point,Operation);
Operation = TDragOperation::Copy;
}
void __fastcall TForm1::DragDrop(const Fmx::Types::TDragObject &Data, const System::Types::TPointF &Point)
{
TForm::DragDrop(Data,Point);
for( int i = Data.Files.Low ; i <= Data.Files.High ; i ++ )
//Memo1->Lines->Add(Data.Files[i]);
Parse_File(Data.Files[i]);
}
__fastcall TForm1::TForm1(TComponent* Owner)
: TForm(Owner)
{
}
長いですが、まだ大丈夫ですかね?そろそろレポジトリの方がいいですかね?パブリックなものも用意したので、ご希望があればurlを公開します。コメントしてください。
以下のプロパティーを持ったjpegファイルをドラグアンドドロップで読み込ませると、
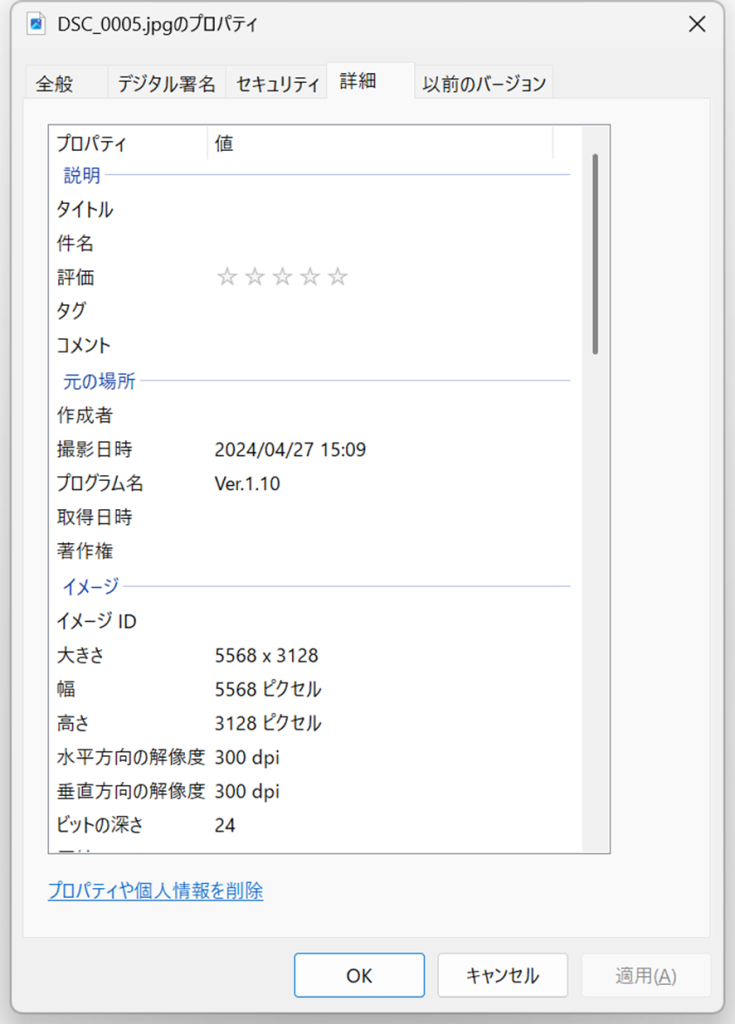
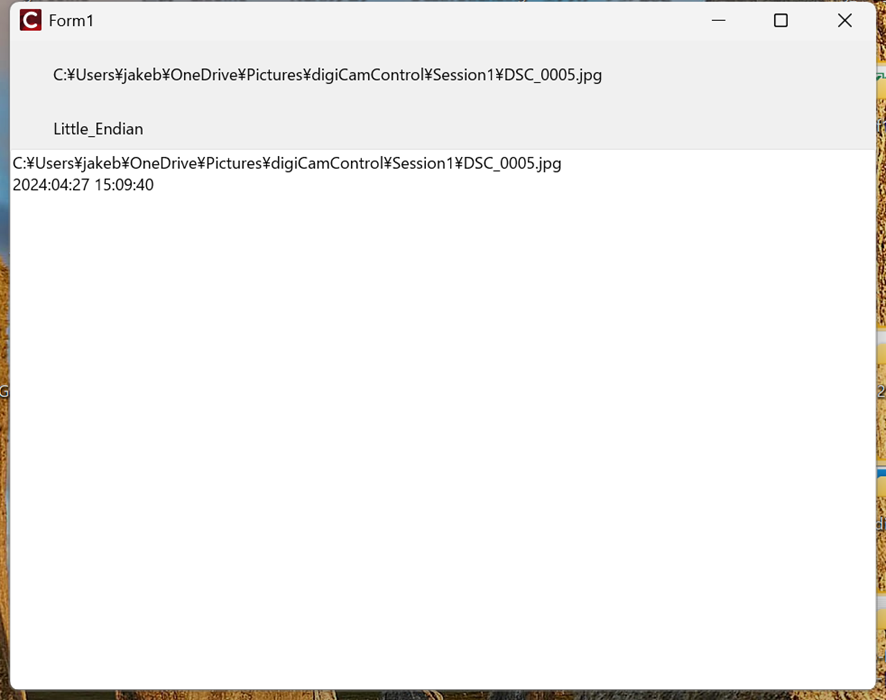
となり、撮影日時が抜き出せています。DSCなんとかかんとかはNikonのカメラのjpegですかね。Little_Endianと認識しています。もひとつ別の例を、
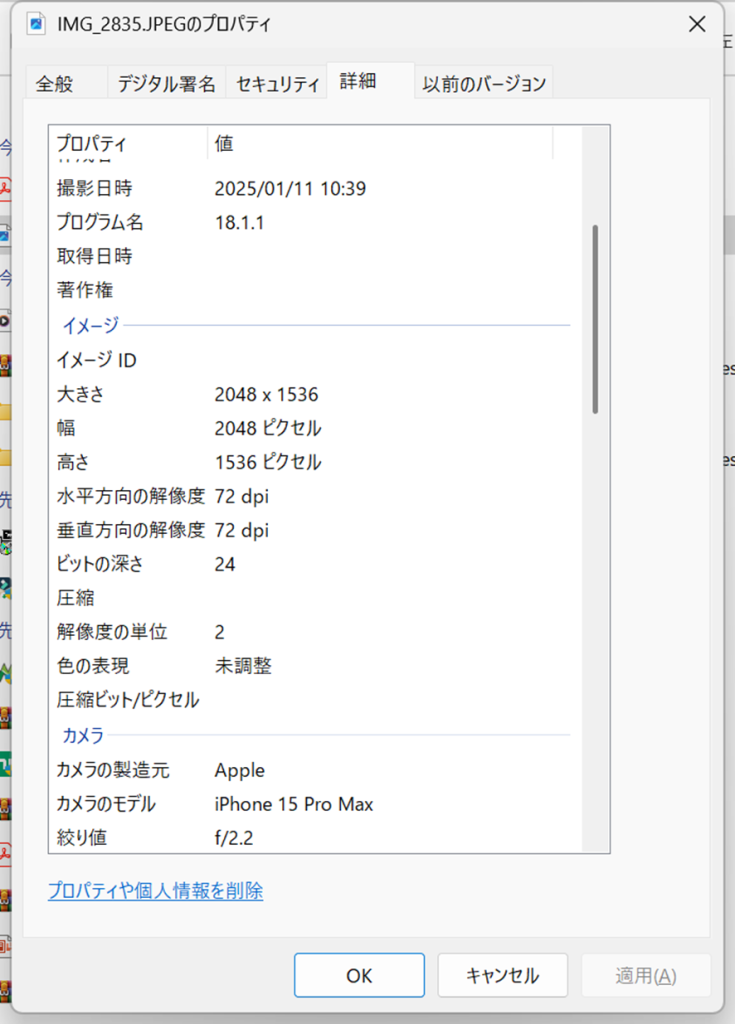
iPhoneで撮影した画像ファイルを落とすと、
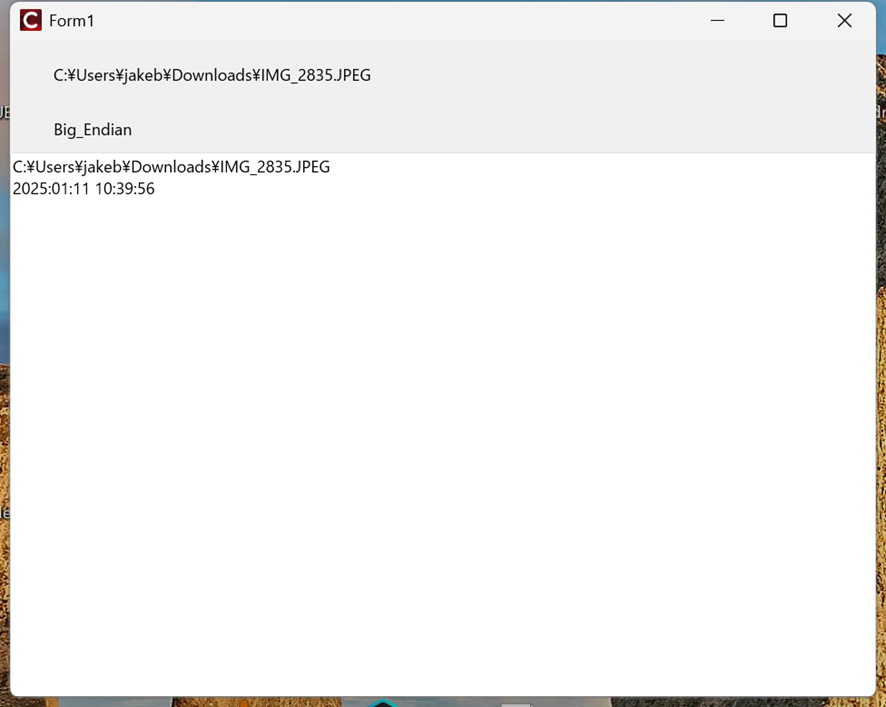
となります。ファイルのタイムスタンプとは違います。
コメント